Over the past few days I’ve put in quite a bit of work on my first iOS game. I’ve tweaked physics, learned how to implement a timer, messed with GameplayKit, and continued to refactor my code so that it’s more clear and efficient.
Yesterday I realized that I hadn’t asked myself a very important question about the game: Is it fun? The answer, as the game currently stands, is no.
So then, the next logical question is: how can I make it fun? Before I attempt to answer that, let me tell you a bit about the game.
Introducing Corgi Corral
I’m planning to call it Corgi Corral. You play as a corgi and your goal is to herd a group of animals (starting with sheep) into their pen. The game is written in Swift, using SpriteKit, and at the moment is accelerometer-controlled. Here’s a very early screenshot:
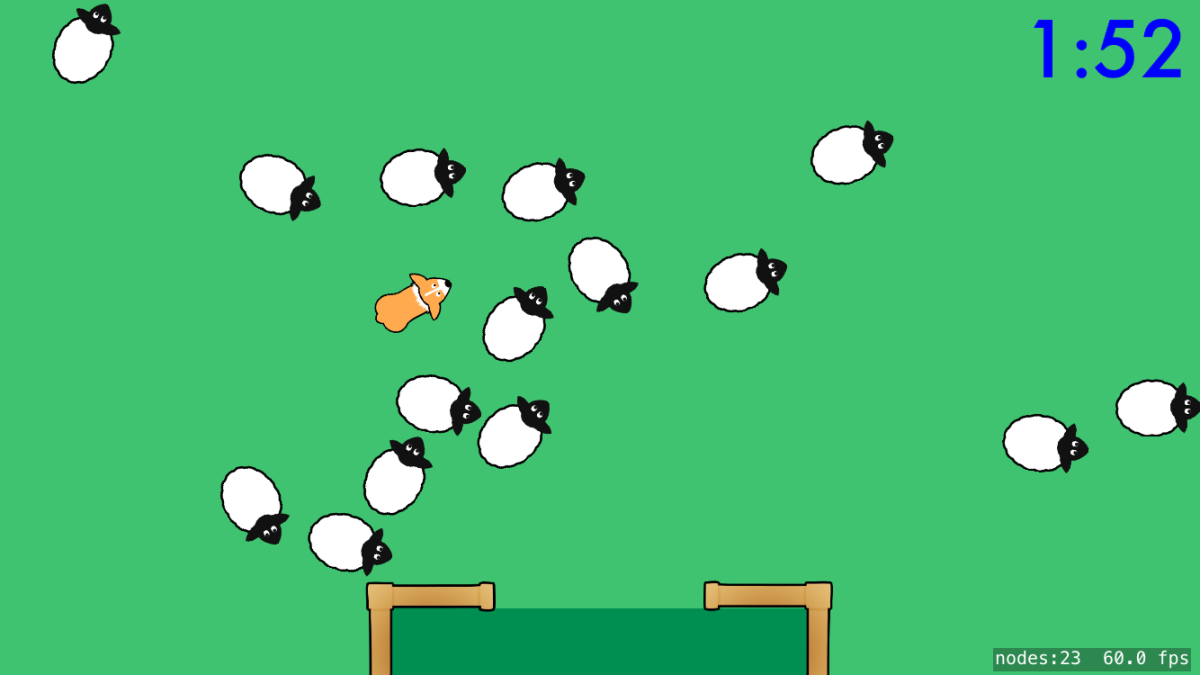
At the moment, the game doesn’t keep score and the sheep aren’t set to flee from the player (instead they just spawn in random positions at the beginning and wander aimlessly). I haven’t set up a lick of collision detection. The timer does nothing except count down. In other words: it’s not even close to being done. But I can already tell that it’s not fun. Funny, maybe, but not fun.
What makes a game fun?
I’m not going to get into Csíkszentmihályi’s theory of flow or the pros and cons of various game mechanics. And obviously, different people find different games fun. But for this game, specifically, I have some ideas.
Challenge. One of the problems with the game as it stands is that there’s not enough skill involved. The accelerometer controls can be a bit unpredictable as can the movements of the sheep, so it’s difficult to make decisions as a player that might improve your score. Therefore, tweaking the responsiveness of both the player and the sheep needs to be a priority. While some randomness in a game is okay, it’s frustrating as a player when you feel like you can’t change your technique to effect a better outcome.
Additionally, I’m torn as to what method of time-based gameplay to use. Should I set a time limit and count down, and see how many sheep the player can get safely into their pen in that amount of time? Or should I start at zero and count up, requiring the player to herd all the sheep into the pen and then awarding them a score based on how long it took? Feedback on that is welcome.
Personality. When I got the idea for Corgi Corral, I pictured it as a somewhat serious game. After all, corgis are actually herding dogs and this is something they used to do for a job. As I began working on the game, however, I changed my mind completely. I want the game to be zany and frantic and silly. I want it to make people laugh.
How can I achieve that? Having a time-based game already introduces an element of franticness, but I can do more. Music and sound effects will help. Some goofy physics and cute animations might also do the trick.
What if the corgi had a little cape? What if there were power ups? What if one level took place in SPACE? I don’t know. All I know is that I need to stay imaginative. After all, corgi-related games remain a mostly unexplored frontier. The possibilities are endless.
Progression. In other words: what makes players want to keep playing? At the moment, I don’t have a good, definitive answer to that. I know that I want Corgi Corral to be a game that people can play while waiting in line. However, I also want to introduce mechanics that make the game a little harder as time goes on. Perhaps there will be a minimum number of sheep that players must herd in order to unlock the next level. The next level might introduce ponds or fire pits or some way to lose sheep while also increasing the minimum number required to progress. Again, feedback and ideas are welcome.
Developing in the open
From this point forward, I plan to post a lot of code snippets and screenshots of the game’s progress. As a beginner, I feel that blogging about this experience (the good and the bad) will not only benefit other beginners but will help me learn as well.
I can’t say for certain how often I’ll post, and this whole idea might get tossed aside for awhile when my son is born (from what I hear, having a newborn can be a bit time-consuming!). I’ll do my best!